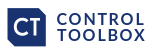 |
- 3.0.1 core module.
|
Go to the documentation of this file. 40 #ifndef GMOCK_INCLUDE_GMOCK_GMOCK_GENERATED_MATCHERS_H_ 41 #define GMOCK_INCLUDE_GMOCK_GMOCK_GENERATED_MATCHERS_H_ 266 #define MATCHER(name, description)\ 267 class name##Matcher {\ 269 template <typename arg_type>\ 270 class gmock_Impl : public ::testing::MatcherInterface<\ 271 GTEST_REFERENCE_TO_CONST_(arg_type)> {\ 275 virtual bool MatchAndExplain(\ 276 GTEST_REFERENCE_TO_CONST_(arg_type) arg,\ 277 ::testing::MatchResultListener* result_listener) const;\ 278 virtual void DescribeTo(::std::ostream* gmock_os) const {\ 279 *gmock_os << FormatDescription(false);\ 281 virtual void DescribeNegationTo(::std::ostream* gmock_os) const {\ 282 *gmock_os << FormatDescription(true);\ 285 ::std::string FormatDescription(bool negation) const {\ 286 ::std::string gmock_description = (description);\ 287 if (!gmock_description.empty()) {\ 288 return gmock_description;\ 290 return ::testing::internal::FormatMatcherDescription(\ 292 ::testing::internal::UniversalTersePrintTupleFieldsToStrings(\ 296 template <typename arg_type>\ 297 operator ::testing::Matcher<arg_type>() const {\ 298 return ::testing::Matcher<arg_type>(\ 299 new gmock_Impl<arg_type>());\ 305 inline name##Matcher name() {\ 306 return name##Matcher();\ 308 template <typename arg_type>\ 309 bool name##Matcher::gmock_Impl<arg_type>::MatchAndExplain(\ 310 GTEST_REFERENCE_TO_CONST_(arg_type) arg,\ 311 ::testing::MatchResultListener* result_listener GTEST_ATTRIBUTE_UNUSED_)\ 314 #define MATCHER_P(name, p0, description)\ 315 template <typename p0##_type>\ 316 class name##MatcherP {\ 318 template <typename arg_type>\ 319 class gmock_Impl : public ::testing::MatcherInterface<\ 320 GTEST_REFERENCE_TO_CONST_(arg_type)> {\ 322 explicit gmock_Impl(p0##_type gmock_p0)\ 323 : p0(::std::move(gmock_p0)) {}\ 324 virtual bool MatchAndExplain(\ 325 GTEST_REFERENCE_TO_CONST_(arg_type) arg,\ 326 ::testing::MatchResultListener* result_listener) const;\ 327 virtual void DescribeTo(::std::ostream* gmock_os) const {\ 328 *gmock_os << FormatDescription(false);\ 330 virtual void DescribeNegationTo(::std::ostream* gmock_os) const {\ 331 *gmock_os << FormatDescription(true);\ 335 ::std::string FormatDescription(bool negation) const {\ 336 ::std::string gmock_description = (description);\ 337 if (!gmock_description.empty()) {\ 338 return gmock_description;\ 340 return ::testing::internal::FormatMatcherDescription(\ 342 ::testing::internal::UniversalTersePrintTupleFieldsToStrings(\ 343 ::std::tuple<p0##_type>(p0)));\ 346 template <typename arg_type>\ 347 operator ::testing::Matcher<arg_type>() const {\ 348 return ::testing::Matcher<arg_type>(\ 349 new gmock_Impl<arg_type>(p0));\ 351 explicit name##MatcherP(p0##_type gmock_p0) : p0(::std::move(gmock_p0)) {\ 356 template <typename p0##_type>\ 357 inline name##MatcherP<p0##_type> name(p0##_type p0) {\ 358 return name##MatcherP<p0##_type>(p0);\ 360 template <typename p0##_type>\ 361 template <typename arg_type>\ 362 bool name##MatcherP<p0##_type>::gmock_Impl<arg_type>::MatchAndExplain(\ 363 GTEST_REFERENCE_TO_CONST_(arg_type) arg,\ 364 ::testing::MatchResultListener* result_listener GTEST_ATTRIBUTE_UNUSED_)\ 367 #define MATCHER_P2(name, p0, p1, description)\ 368 template <typename p0##_type, typename p1##_type>\ 369 class name##MatcherP2 {\ 371 template <typename arg_type>\ 372 class gmock_Impl : public ::testing::MatcherInterface<\ 373 GTEST_REFERENCE_TO_CONST_(arg_type)> {\ 375 gmock_Impl(p0##_type gmock_p0, p1##_type gmock_p1)\ 376 : p0(::std::move(gmock_p0)), p1(::std::move(gmock_p1)) {}\ 377 virtual bool MatchAndExplain(\ 378 GTEST_REFERENCE_TO_CONST_(arg_type) arg,\ 379 ::testing::MatchResultListener* result_listener) const;\ 380 virtual void DescribeTo(::std::ostream* gmock_os) const {\ 381 *gmock_os << FormatDescription(false);\ 383 virtual void DescribeNegationTo(::std::ostream* gmock_os) const {\ 384 *gmock_os << FormatDescription(true);\ 389 ::std::string FormatDescription(bool negation) const {\ 390 ::std::string gmock_description = (description);\ 391 if (!gmock_description.empty()) {\ 392 return gmock_description;\ 394 return ::testing::internal::FormatMatcherDescription(\ 396 ::testing::internal::UniversalTersePrintTupleFieldsToStrings(\ 397 ::std::tuple<p0##_type, p1##_type>(p0, p1)));\ 400 template <typename arg_type>\ 401 operator ::testing::Matcher<arg_type>() const {\ 402 return ::testing::Matcher<arg_type>(\ 403 new gmock_Impl<arg_type>(p0, p1));\ 405 name##MatcherP2(p0##_type gmock_p0, \ 406 p1##_type gmock_p1) : p0(::std::move(gmock_p0)), \ 407 p1(::std::move(gmock_p1)) {\ 413 template <typename p0##_type, typename p1##_type>\ 414 inline name##MatcherP2<p0##_type, p1##_type> name(p0##_type p0, \ 416 return name##MatcherP2<p0##_type, p1##_type>(p0, p1);\ 418 template <typename p0##_type, typename p1##_type>\ 419 template <typename arg_type>\ 420 bool name##MatcherP2<p0##_type, \ 421 p1##_type>::gmock_Impl<arg_type>::MatchAndExplain(\ 422 GTEST_REFERENCE_TO_CONST_(arg_type) arg,\ 423 ::testing::MatchResultListener* result_listener GTEST_ATTRIBUTE_UNUSED_)\ 426 #define MATCHER_P3(name, p0, p1, p2, description)\ 427 template <typename p0##_type, typename p1##_type, typename p2##_type>\ 428 class name##MatcherP3 {\ 430 template <typename arg_type>\ 431 class gmock_Impl : public ::testing::MatcherInterface<\ 432 GTEST_REFERENCE_TO_CONST_(arg_type)> {\ 434 gmock_Impl(p0##_type gmock_p0, p1##_type gmock_p1, p2##_type gmock_p2)\ 435 : p0(::std::move(gmock_p0)), p1(::std::move(gmock_p1)), \ 436 p2(::std::move(gmock_p2)) {}\ 437 virtual bool MatchAndExplain(\ 438 GTEST_REFERENCE_TO_CONST_(arg_type) arg,\ 439 ::testing::MatchResultListener* result_listener) const;\ 440 virtual void DescribeTo(::std::ostream* gmock_os) const {\ 441 *gmock_os << FormatDescription(false);\ 443 virtual void DescribeNegationTo(::std::ostream* gmock_os) const {\ 444 *gmock_os << FormatDescription(true);\ 450 ::std::string FormatDescription(bool negation) const {\ 451 ::std::string gmock_description = (description);\ 452 if (!gmock_description.empty()) {\ 453 return gmock_description;\ 455 return ::testing::internal::FormatMatcherDescription(\ 457 ::testing::internal::UniversalTersePrintTupleFieldsToStrings(\ 458 ::std::tuple<p0##_type, p1##_type, p2##_type>(p0, p1, p2)));\ 461 template <typename arg_type>\ 462 operator ::testing::Matcher<arg_type>() const {\ 463 return ::testing::Matcher<arg_type>(\ 464 new gmock_Impl<arg_type>(p0, p1, p2));\ 466 name##MatcherP3(p0##_type gmock_p0, p1##_type gmock_p1, \ 467 p2##_type gmock_p2) : p0(::std::move(gmock_p0)), \ 468 p1(::std::move(gmock_p1)), p2(::std::move(gmock_p2)) {\ 475 template <typename p0##_type, typename p1##_type, typename p2##_type>\ 476 inline name##MatcherP3<p0##_type, p1##_type, p2##_type> name(p0##_type p0, \ 477 p1##_type p1, p2##_type p2) {\ 478 return name##MatcherP3<p0##_type, p1##_type, p2##_type>(p0, p1, p2);\ 480 template <typename p0##_type, typename p1##_type, typename p2##_type>\ 481 template <typename arg_type>\ 482 bool name##MatcherP3<p0##_type, p1##_type, \ 483 p2##_type>::gmock_Impl<arg_type>::MatchAndExplain(\ 484 GTEST_REFERENCE_TO_CONST_(arg_type) arg,\ 485 ::testing::MatchResultListener* result_listener GTEST_ATTRIBUTE_UNUSED_)\ 488 #define MATCHER_P4(name, p0, p1, p2, p3, description)\ 489 template <typename p0##_type, typename p1##_type, typename p2##_type, \ 491 class name##MatcherP4 {\ 493 template <typename arg_type>\ 494 class gmock_Impl : public ::testing::MatcherInterface<\ 495 GTEST_REFERENCE_TO_CONST_(arg_type)> {\ 497 gmock_Impl(p0##_type gmock_p0, p1##_type gmock_p1, p2##_type gmock_p2, \ 499 : p0(::std::move(gmock_p0)), p1(::std::move(gmock_p1)), \ 500 p2(::std::move(gmock_p2)), p3(::std::move(gmock_p3)) {}\ 501 virtual bool MatchAndExplain(\ 502 GTEST_REFERENCE_TO_CONST_(arg_type) arg,\ 503 ::testing::MatchResultListener* result_listener) const;\ 504 virtual void DescribeTo(::std::ostream* gmock_os) const {\ 505 *gmock_os << FormatDescription(false);\ 507 virtual void DescribeNegationTo(::std::ostream* gmock_os) const {\ 508 *gmock_os << FormatDescription(true);\ 515 ::std::string FormatDescription(bool negation) const {\ 516 ::std::string gmock_description = (description);\ 517 if (!gmock_description.empty()) {\ 518 return gmock_description;\ 520 return ::testing::internal::FormatMatcherDescription(\ 522 ::testing::internal::UniversalTersePrintTupleFieldsToStrings(\ 523 ::std::tuple<p0##_type, p1##_type, p2##_type, p3##_type>(p0, \ 527 template <typename arg_type>\ 528 operator ::testing::Matcher<arg_type>() const {\ 529 return ::testing::Matcher<arg_type>(\ 530 new gmock_Impl<arg_type>(p0, p1, p2, p3));\ 532 name##MatcherP4(p0##_type gmock_p0, p1##_type gmock_p1, \ 533 p2##_type gmock_p2, p3##_type gmock_p3) : p0(::std::move(gmock_p0)), \ 534 p1(::std::move(gmock_p1)), p2(::std::move(gmock_p2)), \ 535 p3(::std::move(gmock_p3)) {\ 543 template <typename p0##_type, typename p1##_type, typename p2##_type, \ 545 inline name##MatcherP4<p0##_type, p1##_type, p2##_type, \ 546 p3##_type> name(p0##_type p0, p1##_type p1, p2##_type p2, \ 548 return name##MatcherP4<p0##_type, p1##_type, p2##_type, p3##_type>(p0, \ 551 template <typename p0##_type, typename p1##_type, typename p2##_type, \ 553 template <typename arg_type>\ 554 bool name##MatcherP4<p0##_type, p1##_type, p2##_type, \ 555 p3##_type>::gmock_Impl<arg_type>::MatchAndExplain(\ 556 GTEST_REFERENCE_TO_CONST_(arg_type) arg,\ 557 ::testing::MatchResultListener* result_listener GTEST_ATTRIBUTE_UNUSED_)\ 560 #define MATCHER_P5(name, p0, p1, p2, p3, p4, description)\ 561 template <typename p0##_type, typename p1##_type, typename p2##_type, \ 562 typename p3##_type, typename p4##_type>\ 563 class name##MatcherP5 {\ 565 template <typename arg_type>\ 566 class gmock_Impl : public ::testing::MatcherInterface<\ 567 GTEST_REFERENCE_TO_CONST_(arg_type)> {\ 569 gmock_Impl(p0##_type gmock_p0, p1##_type gmock_p1, p2##_type gmock_p2, \ 570 p3##_type gmock_p3, p4##_type gmock_p4)\ 571 : p0(::std::move(gmock_p0)), p1(::std::move(gmock_p1)), \ 572 p2(::std::move(gmock_p2)), p3(::std::move(gmock_p3)), \ 573 p4(::std::move(gmock_p4)) {}\ 574 virtual bool MatchAndExplain(\ 575 GTEST_REFERENCE_TO_CONST_(arg_type) arg,\ 576 ::testing::MatchResultListener* result_listener) const;\ 577 virtual void DescribeTo(::std::ostream* gmock_os) const {\ 578 *gmock_os << FormatDescription(false);\ 580 virtual void DescribeNegationTo(::std::ostream* gmock_os) const {\ 581 *gmock_os << FormatDescription(true);\ 589 ::std::string FormatDescription(bool negation) const {\ 590 ::std::string gmock_description = (description);\ 591 if (!gmock_description.empty()) {\ 592 return gmock_description;\ 594 return ::testing::internal::FormatMatcherDescription(\ 596 ::testing::internal::UniversalTersePrintTupleFieldsToStrings(\ 597 ::std::tuple<p0##_type, p1##_type, p2##_type, p3##_type, \ 598 p4##_type>(p0, p1, p2, p3, p4)));\ 601 template <typename arg_type>\ 602 operator ::testing::Matcher<arg_type>() const {\ 603 return ::testing::Matcher<arg_type>(\ 604 new gmock_Impl<arg_type>(p0, p1, p2, p3, p4));\ 606 name##MatcherP5(p0##_type gmock_p0, p1##_type gmock_p1, \ 607 p2##_type gmock_p2, p3##_type gmock_p3, \ 608 p4##_type gmock_p4) : p0(::std::move(gmock_p0)), \ 609 p1(::std::move(gmock_p1)), p2(::std::move(gmock_p2)), \ 610 p3(::std::move(gmock_p3)), p4(::std::move(gmock_p4)) {\ 619 template <typename p0##_type, typename p1##_type, typename p2##_type, \ 620 typename p3##_type, typename p4##_type>\ 621 inline name##MatcherP5<p0##_type, p1##_type, p2##_type, p3##_type, \ 622 p4##_type> name(p0##_type p0, p1##_type p1, p2##_type p2, p3##_type p3, \ 624 return name##MatcherP5<p0##_type, p1##_type, p2##_type, p3##_type, \ 625 p4##_type>(p0, p1, p2, p3, p4);\ 627 template <typename p0##_type, typename p1##_type, typename p2##_type, \ 628 typename p3##_type, typename p4##_type>\ 629 template <typename arg_type>\ 630 bool name##MatcherP5<p0##_type, p1##_type, p2##_type, p3##_type, \ 631 p4##_type>::gmock_Impl<arg_type>::MatchAndExplain(\ 632 GTEST_REFERENCE_TO_CONST_(arg_type) arg,\ 633 ::testing::MatchResultListener* result_listener GTEST_ATTRIBUTE_UNUSED_)\ 636 #define MATCHER_P6(name, p0, p1, p2, p3, p4, p5, description)\ 637 template <typename p0##_type, typename p1##_type, typename p2##_type, \ 638 typename p3##_type, typename p4##_type, typename p5##_type>\ 639 class name##MatcherP6 {\ 641 template <typename arg_type>\ 642 class gmock_Impl : public ::testing::MatcherInterface<\ 643 GTEST_REFERENCE_TO_CONST_(arg_type)> {\ 645 gmock_Impl(p0##_type gmock_p0, p1##_type gmock_p1, p2##_type gmock_p2, \ 646 p3##_type gmock_p3, p4##_type gmock_p4, p5##_type gmock_p5)\ 647 : p0(::std::move(gmock_p0)), p1(::std::move(gmock_p1)), \ 648 p2(::std::move(gmock_p2)), p3(::std::move(gmock_p3)), \ 649 p4(::std::move(gmock_p4)), p5(::std::move(gmock_p5)) {}\ 650 virtual bool MatchAndExplain(\ 651 GTEST_REFERENCE_TO_CONST_(arg_type) arg,\ 652 ::testing::MatchResultListener* result_listener) const;\ 653 virtual void DescribeTo(::std::ostream* gmock_os) const {\ 654 *gmock_os << FormatDescription(false);\ 656 virtual void DescribeNegationTo(::std::ostream* gmock_os) const {\ 657 *gmock_os << FormatDescription(true);\ 666 ::std::string FormatDescription(bool negation) const {\ 667 ::std::string gmock_description = (description);\ 668 if (!gmock_description.empty()) {\ 669 return gmock_description;\ 671 return ::testing::internal::FormatMatcherDescription(\ 673 ::testing::internal::UniversalTersePrintTupleFieldsToStrings(\ 674 ::std::tuple<p0##_type, p1##_type, p2##_type, p3##_type, \ 675 p4##_type, p5##_type>(p0, p1, p2, p3, p4, p5)));\ 678 template <typename arg_type>\ 679 operator ::testing::Matcher<arg_type>() const {\ 680 return ::testing::Matcher<arg_type>(\ 681 new gmock_Impl<arg_type>(p0, p1, p2, p3, p4, p5));\ 683 name##MatcherP6(p0##_type gmock_p0, p1##_type gmock_p1, \ 684 p2##_type gmock_p2, p3##_type gmock_p3, p4##_type gmock_p4, \ 685 p5##_type gmock_p5) : p0(::std::move(gmock_p0)), \ 686 p1(::std::move(gmock_p1)), p2(::std::move(gmock_p2)), \ 687 p3(::std::move(gmock_p3)), p4(::std::move(gmock_p4)), \ 688 p5(::std::move(gmock_p5)) {\ 698 template <typename p0##_type, typename p1##_type, typename p2##_type, \ 699 typename p3##_type, typename p4##_type, typename p5##_type>\ 700 inline name##MatcherP6<p0##_type, p1##_type, p2##_type, p3##_type, \ 701 p4##_type, p5##_type> name(p0##_type p0, p1##_type p1, p2##_type p2, \ 702 p3##_type p3, p4##_type p4, p5##_type p5) {\ 703 return name##MatcherP6<p0##_type, p1##_type, p2##_type, p3##_type, \ 704 p4##_type, p5##_type>(p0, p1, p2, p3, p4, p5);\ 706 template <typename p0##_type, typename p1##_type, typename p2##_type, \ 707 typename p3##_type, typename p4##_type, typename p5##_type>\ 708 template <typename arg_type>\ 709 bool name##MatcherP6<p0##_type, p1##_type, p2##_type, p3##_type, p4##_type, \ 710 p5##_type>::gmock_Impl<arg_type>::MatchAndExplain(\ 711 GTEST_REFERENCE_TO_CONST_(arg_type) arg,\ 712 ::testing::MatchResultListener* result_listener GTEST_ATTRIBUTE_UNUSED_)\ 715 #define MATCHER_P7(name, p0, p1, p2, p3, p4, p5, p6, description)\ 716 template <typename p0##_type, typename p1##_type, typename p2##_type, \ 717 typename p3##_type, typename p4##_type, typename p5##_type, \ 719 class name##MatcherP7 {\ 721 template <typename arg_type>\ 722 class gmock_Impl : public ::testing::MatcherInterface<\ 723 GTEST_REFERENCE_TO_CONST_(arg_type)> {\ 725 gmock_Impl(p0##_type gmock_p0, p1##_type gmock_p1, p2##_type gmock_p2, \ 726 p3##_type gmock_p3, p4##_type gmock_p4, p5##_type gmock_p5, \ 728 : p0(::std::move(gmock_p0)), p1(::std::move(gmock_p1)), \ 729 p2(::std::move(gmock_p2)), p3(::std::move(gmock_p3)), \ 730 p4(::std::move(gmock_p4)), p5(::std::move(gmock_p5)), \ 731 p6(::std::move(gmock_p6)) {}\ 732 virtual bool MatchAndExplain(\ 733 GTEST_REFERENCE_TO_CONST_(arg_type) arg,\ 734 ::testing::MatchResultListener* result_listener) const;\ 735 virtual void DescribeTo(::std::ostream* gmock_os) const {\ 736 *gmock_os << FormatDescription(false);\ 738 virtual void DescribeNegationTo(::std::ostream* gmock_os) const {\ 739 *gmock_os << FormatDescription(true);\ 749 ::std::string FormatDescription(bool negation) const {\ 750 ::std::string gmock_description = (description);\ 751 if (!gmock_description.empty()) {\ 752 return gmock_description;\ 754 return ::testing::internal::FormatMatcherDescription(\ 756 ::testing::internal::UniversalTersePrintTupleFieldsToStrings(\ 757 ::std::tuple<p0##_type, p1##_type, p2##_type, p3##_type, \ 758 p4##_type, p5##_type, p6##_type>(p0, p1, p2, p3, p4, p5, \ 762 template <typename arg_type>\ 763 operator ::testing::Matcher<arg_type>() const {\ 764 return ::testing::Matcher<arg_type>(\ 765 new gmock_Impl<arg_type>(p0, p1, p2, p3, p4, p5, p6));\ 767 name##MatcherP7(p0##_type gmock_p0, p1##_type gmock_p1, \ 768 p2##_type gmock_p2, p3##_type gmock_p3, p4##_type gmock_p4, \ 769 p5##_type gmock_p5, p6##_type gmock_p6) : p0(::std::move(gmock_p0)), \ 770 p1(::std::move(gmock_p1)), p2(::std::move(gmock_p2)), \ 771 p3(::std::move(gmock_p3)), p4(::std::move(gmock_p4)), \ 772 p5(::std::move(gmock_p5)), p6(::std::move(gmock_p6)) {\ 783 template <typename p0##_type, typename p1##_type, typename p2##_type, \ 784 typename p3##_type, typename p4##_type, typename p5##_type, \ 786 inline name##MatcherP7<p0##_type, p1##_type, p2##_type, p3##_type, \ 787 p4##_type, p5##_type, p6##_type> name(p0##_type p0, p1##_type p1, \ 788 p2##_type p2, p3##_type p3, p4##_type p4, p5##_type p5, \ 790 return name##MatcherP7<p0##_type, p1##_type, p2##_type, p3##_type, \ 791 p4##_type, p5##_type, p6##_type>(p0, p1, p2, p3, p4, p5, p6);\ 793 template <typename p0##_type, typename p1##_type, typename p2##_type, \ 794 typename p3##_type, typename p4##_type, typename p5##_type, \ 796 template <typename arg_type>\ 797 bool name##MatcherP7<p0##_type, p1##_type, p2##_type, p3##_type, p4##_type, \ 798 p5##_type, p6##_type>::gmock_Impl<arg_type>::MatchAndExplain(\ 799 GTEST_REFERENCE_TO_CONST_(arg_type) arg,\ 800 ::testing::MatchResultListener* result_listener GTEST_ATTRIBUTE_UNUSED_)\ 803 #define MATCHER_P8(name, p0, p1, p2, p3, p4, p5, p6, p7, description)\ 804 template <typename p0##_type, typename p1##_type, typename p2##_type, \ 805 typename p3##_type, typename p4##_type, typename p5##_type, \ 806 typename p6##_type, typename p7##_type>\ 807 class name##MatcherP8 {\ 809 template <typename arg_type>\ 810 class gmock_Impl : public ::testing::MatcherInterface<\ 811 GTEST_REFERENCE_TO_CONST_(arg_type)> {\ 813 gmock_Impl(p0##_type gmock_p0, p1##_type gmock_p1, p2##_type gmock_p2, \ 814 p3##_type gmock_p3, p4##_type gmock_p4, p5##_type gmock_p5, \ 815 p6##_type gmock_p6, p7##_type gmock_p7)\ 816 : p0(::std::move(gmock_p0)), p1(::std::move(gmock_p1)), \ 817 p2(::std::move(gmock_p2)), p3(::std::move(gmock_p3)), \ 818 p4(::std::move(gmock_p4)), p5(::std::move(gmock_p5)), \ 819 p6(::std::move(gmock_p6)), p7(::std::move(gmock_p7)) {}\ 820 virtual bool MatchAndExplain(\ 821 GTEST_REFERENCE_TO_CONST_(arg_type) arg,\ 822 ::testing::MatchResultListener* result_listener) const;\ 823 virtual void DescribeTo(::std::ostream* gmock_os) const {\ 824 *gmock_os << FormatDescription(false);\ 826 virtual void DescribeNegationTo(::std::ostream* gmock_os) const {\ 827 *gmock_os << FormatDescription(true);\ 838 ::std::string FormatDescription(bool negation) const {\ 839 ::std::string gmock_description = (description);\ 840 if (!gmock_description.empty()) {\ 841 return gmock_description;\ 843 return ::testing::internal::FormatMatcherDescription(\ 845 ::testing::internal::UniversalTersePrintTupleFieldsToStrings(\ 846 ::std::tuple<p0##_type, p1##_type, p2##_type, p3##_type, \ 847 p4##_type, p5##_type, p6##_type, p7##_type>(p0, p1, p2, \ 848 p3, p4, p5, p6, p7)));\ 851 template <typename arg_type>\ 852 operator ::testing::Matcher<arg_type>() const {\ 853 return ::testing::Matcher<arg_type>(\ 854 new gmock_Impl<arg_type>(p0, p1, p2, p3, p4, p5, p6, p7));\ 856 name##MatcherP8(p0##_type gmock_p0, p1##_type gmock_p1, \ 857 p2##_type gmock_p2, p3##_type gmock_p3, p4##_type gmock_p4, \ 858 p5##_type gmock_p5, p6##_type gmock_p6, \ 859 p7##_type gmock_p7) : p0(::std::move(gmock_p0)), \ 860 p1(::std::move(gmock_p1)), p2(::std::move(gmock_p2)), \ 861 p3(::std::move(gmock_p3)), p4(::std::move(gmock_p4)), \ 862 p5(::std::move(gmock_p5)), p6(::std::move(gmock_p6)), \ 863 p7(::std::move(gmock_p7)) {\ 875 template <typename p0##_type, typename p1##_type, typename p2##_type, \ 876 typename p3##_type, typename p4##_type, typename p5##_type, \ 877 typename p6##_type, typename p7##_type>\ 878 inline name##MatcherP8<p0##_type, p1##_type, p2##_type, p3##_type, \ 879 p4##_type, p5##_type, p6##_type, p7##_type> name(p0##_type p0, \ 880 p1##_type p1, p2##_type p2, p3##_type p3, p4##_type p4, p5##_type p5, \ 881 p6##_type p6, p7##_type p7) {\ 882 return name##MatcherP8<p0##_type, p1##_type, p2##_type, p3##_type, \ 883 p4##_type, p5##_type, p6##_type, p7##_type>(p0, p1, p2, p3, p4, p5, \ 886 template <typename p0##_type, typename p1##_type, typename p2##_type, \ 887 typename p3##_type, typename p4##_type, typename p5##_type, \ 888 typename p6##_type, typename p7##_type>\ 889 template <typename arg_type>\ 890 bool name##MatcherP8<p0##_type, p1##_type, p2##_type, p3##_type, p4##_type, \ 891 p5##_type, p6##_type, \ 892 p7##_type>::gmock_Impl<arg_type>::MatchAndExplain(\ 893 GTEST_REFERENCE_TO_CONST_(arg_type) arg,\ 894 ::testing::MatchResultListener* result_listener GTEST_ATTRIBUTE_UNUSED_)\ 897 #define MATCHER_P9(name, p0, p1, p2, p3, p4, p5, p6, p7, p8, description)\ 898 template <typename p0##_type, typename p1##_type, typename p2##_type, \ 899 typename p3##_type, typename p4##_type, typename p5##_type, \ 900 typename p6##_type, typename p7##_type, typename p8##_type>\ 901 class name##MatcherP9 {\ 903 template <typename arg_type>\ 904 class gmock_Impl : public ::testing::MatcherInterface<\ 905 GTEST_REFERENCE_TO_CONST_(arg_type)> {\ 907 gmock_Impl(p0##_type gmock_p0, p1##_type gmock_p1, p2##_type gmock_p2, \ 908 p3##_type gmock_p3, p4##_type gmock_p4, p5##_type gmock_p5, \ 909 p6##_type gmock_p6, p7##_type gmock_p7, p8##_type gmock_p8)\ 910 : p0(::std::move(gmock_p0)), p1(::std::move(gmock_p1)), \ 911 p2(::std::move(gmock_p2)), p3(::std::move(gmock_p3)), \ 912 p4(::std::move(gmock_p4)), p5(::std::move(gmock_p5)), \ 913 p6(::std::move(gmock_p6)), p7(::std::move(gmock_p7)), \ 914 p8(::std::move(gmock_p8)) {}\ 915 virtual bool MatchAndExplain(\ 916 GTEST_REFERENCE_TO_CONST_(arg_type) arg,\ 917 ::testing::MatchResultListener* result_listener) const;\ 918 virtual void DescribeTo(::std::ostream* gmock_os) const {\ 919 *gmock_os << FormatDescription(false);\ 921 virtual void DescribeNegationTo(::std::ostream* gmock_os) const {\ 922 *gmock_os << FormatDescription(true);\ 934 ::std::string FormatDescription(bool negation) const {\ 935 ::std::string gmock_description = (description);\ 936 if (!gmock_description.empty()) {\ 937 return gmock_description;\ 939 return ::testing::internal::FormatMatcherDescription(\ 941 ::testing::internal::UniversalTersePrintTupleFieldsToStrings(\ 942 ::std::tuple<p0##_type, p1##_type, p2##_type, p3##_type, \ 943 p4##_type, p5##_type, p6##_type, p7##_type, \ 944 p8##_type>(p0, p1, p2, p3, p4, p5, p6, p7, p8)));\ 947 template <typename arg_type>\ 948 operator ::testing::Matcher<arg_type>() const {\ 949 return ::testing::Matcher<arg_type>(\ 950 new gmock_Impl<arg_type>(p0, p1, p2, p3, p4, p5, p6, p7, p8));\ 952 name##MatcherP9(p0##_type gmock_p0, p1##_type gmock_p1, \ 953 p2##_type gmock_p2, p3##_type gmock_p3, p4##_type gmock_p4, \ 954 p5##_type gmock_p5, p6##_type gmock_p6, p7##_type gmock_p7, \ 955 p8##_type gmock_p8) : p0(::std::move(gmock_p0)), \ 956 p1(::std::move(gmock_p1)), p2(::std::move(gmock_p2)), \ 957 p3(::std::move(gmock_p3)), p4(::std::move(gmock_p4)), \ 958 p5(::std::move(gmock_p5)), p6(::std::move(gmock_p6)), \ 959 p7(::std::move(gmock_p7)), p8(::std::move(gmock_p8)) {\ 972 template <typename p0##_type, typename p1##_type, typename p2##_type, \ 973 typename p3##_type, typename p4##_type, typename p5##_type, \ 974 typename p6##_type, typename p7##_type, typename p8##_type>\ 975 inline name##MatcherP9<p0##_type, p1##_type, p2##_type, p3##_type, \ 976 p4##_type, p5##_type, p6##_type, p7##_type, \ 977 p8##_type> name(p0##_type p0, p1##_type p1, p2##_type p2, p3##_type p3, \ 978 p4##_type p4, p5##_type p5, p6##_type p6, p7##_type p7, \ 980 return name##MatcherP9<p0##_type, p1##_type, p2##_type, p3##_type, \ 981 p4##_type, p5##_type, p6##_type, p7##_type, p8##_type>(p0, p1, p2, \ 982 p3, p4, p5, p6, p7, p8);\ 984 template <typename p0##_type, typename p1##_type, typename p2##_type, \ 985 typename p3##_type, typename p4##_type, typename p5##_type, \ 986 typename p6##_type, typename p7##_type, typename p8##_type>\ 987 template <typename arg_type>\ 988 bool name##MatcherP9<p0##_type, p1##_type, p2##_type, p3##_type, p4##_type, \ 989 p5##_type, p6##_type, p7##_type, \ 990 p8##_type>::gmock_Impl<arg_type>::MatchAndExplain(\ 991 GTEST_REFERENCE_TO_CONST_(arg_type) arg,\ 992 ::testing::MatchResultListener* result_listener GTEST_ATTRIBUTE_UNUSED_)\ 995 #define MATCHER_P10(name, p0, p1, p2, p3, p4, p5, p6, p7, p8, p9, description)\ 996 template <typename p0##_type, typename p1##_type, typename p2##_type, \ 997 typename p3##_type, typename p4##_type, typename p5##_type, \ 998 typename p6##_type, typename p7##_type, typename p8##_type, \ 1000 class name##MatcherP10 {\ 1002 template <typename arg_type>\ 1003 class gmock_Impl : public ::testing::MatcherInterface<\ 1004 GTEST_REFERENCE_TO_CONST_(arg_type)> {\ 1006 gmock_Impl(p0##_type gmock_p0, p1##_type gmock_p1, p2##_type gmock_p2, \ 1007 p3##_type gmock_p3, p4##_type gmock_p4, p5##_type gmock_p5, \ 1008 p6##_type gmock_p6, p7##_type gmock_p7, p8##_type gmock_p8, \ 1009 p9##_type gmock_p9)\ 1010 : p0(::std::move(gmock_p0)), p1(::std::move(gmock_p1)), \ 1011 p2(::std::move(gmock_p2)), p3(::std::move(gmock_p3)), \ 1012 p4(::std::move(gmock_p4)), p5(::std::move(gmock_p5)), \ 1013 p6(::std::move(gmock_p6)), p7(::std::move(gmock_p7)), \ 1014 p8(::std::move(gmock_p8)), p9(::std::move(gmock_p9)) {}\ 1015 virtual bool MatchAndExplain(\ 1016 GTEST_REFERENCE_TO_CONST_(arg_type) arg,\ 1017 ::testing::MatchResultListener* result_listener) const;\ 1018 virtual void DescribeTo(::std::ostream* gmock_os) const {\ 1019 *gmock_os << FormatDescription(false);\ 1021 virtual void DescribeNegationTo(::std::ostream* gmock_os) const {\ 1022 *gmock_os << FormatDescription(true);\ 1024 p0##_type const p0;\ 1025 p1##_type const p1;\ 1026 p2##_type const p2;\ 1027 p3##_type const p3;\ 1028 p4##_type const p4;\ 1029 p5##_type const p5;\ 1030 p6##_type const p6;\ 1031 p7##_type const p7;\ 1032 p8##_type const p8;\ 1033 p9##_type const p9;\ 1035 ::std::string FormatDescription(bool negation) const {\ 1036 ::std::string gmock_description = (description);\ 1037 if (!gmock_description.empty()) {\ 1038 return gmock_description;\ 1040 return ::testing::internal::FormatMatcherDescription(\ 1042 ::testing::internal::UniversalTersePrintTupleFieldsToStrings(\ 1043 ::std::tuple<p0##_type, p1##_type, p2##_type, p3##_type, \ 1044 p4##_type, p5##_type, p6##_type, p7##_type, p8##_type, \ 1045 p9##_type>(p0, p1, p2, p3, p4, p5, p6, p7, p8, p9)));\ 1048 template <typename arg_type>\ 1049 operator ::testing::Matcher<arg_type>() const {\ 1050 return ::testing::Matcher<arg_type>(\ 1051 new gmock_Impl<arg_type>(p0, p1, p2, p3, p4, p5, p6, p7, p8, p9));\ 1053 name##MatcherP10(p0##_type gmock_p0, p1##_type gmock_p1, \ 1054 p2##_type gmock_p2, p3##_type gmock_p3, p4##_type gmock_p4, \ 1055 p5##_type gmock_p5, p6##_type gmock_p6, p7##_type gmock_p7, \ 1056 p8##_type gmock_p8, p9##_type gmock_p9) : p0(::std::move(gmock_p0)), \ 1057 p1(::std::move(gmock_p1)), p2(::std::move(gmock_p2)), \ 1058 p3(::std::move(gmock_p3)), p4(::std::move(gmock_p4)), \ 1059 p5(::std::move(gmock_p5)), p6(::std::move(gmock_p6)), \ 1060 p7(::std::move(gmock_p7)), p8(::std::move(gmock_p8)), \ 1061 p9(::std::move(gmock_p9)) {\ 1063 p0##_type const p0;\ 1064 p1##_type const p1;\ 1065 p2##_type const p2;\ 1066 p3##_type const p3;\ 1067 p4##_type const p4;\ 1068 p5##_type const p5;\ 1069 p6##_type const p6;\ 1070 p7##_type const p7;\ 1071 p8##_type const p8;\ 1072 p9##_type const p9;\ 1075 template <typename p0##_type, typename p1##_type, typename p2##_type, \ 1076 typename p3##_type, typename p4##_type, typename p5##_type, \ 1077 typename p6##_type, typename p7##_type, typename p8##_type, \ 1078 typename p9##_type>\ 1079 inline name##MatcherP10<p0##_type, p1##_type, p2##_type, p3##_type, \ 1080 p4##_type, p5##_type, p6##_type, p7##_type, p8##_type, \ 1081 p9##_type> name(p0##_type p0, p1##_type p1, p2##_type p2, p3##_type p3, \ 1082 p4##_type p4, p5##_type p5, p6##_type p6, p7##_type p7, p8##_type p8, \ 1084 return name##MatcherP10<p0##_type, p1##_type, p2##_type, p3##_type, \ 1085 p4##_type, p5##_type, p6##_type, p7##_type, p8##_type, p9##_type>(p0, \ 1086 p1, p2, p3, p4, p5, p6, p7, p8, p9);\ 1088 template <typename p0##_type, typename p1##_type, typename p2##_type, \ 1089 typename p3##_type, typename p4##_type, typename p5##_type, \ 1090 typename p6##_type, typename p7##_type, typename p8##_type, \ 1091 typename p9##_type>\ 1092 template <typename arg_type>\ 1093 bool name##MatcherP10<p0##_type, p1##_type, p2##_type, p3##_type, \ 1094 p4##_type, p5##_type, p6##_type, p7##_type, p8##_type, \ 1095 p9##_type>::gmock_Impl<arg_type>::MatchAndExplain(\ 1096 GTEST_REFERENCE_TO_CONST_(arg_type) arg,\ 1097 ::testing::MatchResultListener* result_listener GTEST_ATTRIBUTE_UNUSED_)\ 1100 #endif // GMOCK_INCLUDE_GMOCK_GMOCK_GENERATED_MATCHERS_H_